Author | Comment |
MufinMcFlufin Probe Posted: 7 Mar 2009 10:04 GMT Total Posts: 1 | Can anyone post some codes on here that will help working programs work much faster? I already know a couple like: -use "Delvar (variable)" instead of "0->(variable)" -don't put end quotes or parenthesis's whenever possible -avoid using labels whenever possible -Try to code If Then statements on a single line via "If (boolean statement):Then" or the "then" could be the single thing you want the If statement to do -Don't do "If x=a:5->x" because the if statement checks for a boolean statement, that means it checks the "x=1" and if true, returns a value of 1. The If statement then does the action if the value of the statement was a 1. So you could shorten it by just putting 5(x=a)->x. This will store 0 into x if false, but will store 5 into x if true. I've found a way to let it keep it's value if false: :5(x=a)+x*not(x=a)->x In this case, if x=a, it will multiply 5 times the boolean true or false, and then add to it the opposite of the same boolean statement multiplied by x and store the total value into x Ex: a=1, x=2 :5(1=2)+2*not(1=2)->x :5(0)+2*not(0)->x :0+2*1->x :2->x Those are all I can remember at the moment other than some that really just apply to certain situations. (using variables for list numbers, simplifying and grouping together statements that return the same value. Ex: :If c=1:a->d :If c=2:d->a :If c=3:a->d :If c=4:d->a Instead of that, do something like this :If c=1 or c=3:a->d :If c=2 or 2=4:d->a
Can anyone else post some things like these that make codes go faster? I want to get my BASIC Tic-Tac-Toe program working faster before I post it up on the internet. |
Xphoenix Ultralisk
 Posted: 7 Mar 2009 21:08 GMT Total Posts: 210 | These minimize the size of the program, but don't necessarily make it run faster. For instance,
:If X :Then :[stuff] :End
is [supposedly] faster than
:If X :[stuff]
if there's only one line of [stuff], if X is false.
There are so many situations that can be optimized, posting them all would be suicide. Try these pages: http://tibasicdev.wikidot.com/optimize-general http://tibasicdev.wikidot.com/optimize-input http://tibasicdev.wikidot.com/optimize-graph http://tibasicdev.wikidot.com/optimize
--- ~Xphoenix |
Vectris Ultralisk
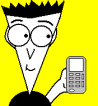 Posted: 11 Mar 2009 15:18 GMT Total Posts: 375 | Why would you avoid Lbls, they can be very useful. Also why would you use DelVar X instead of 0->X?
Anyone know why?
|
haveacalc Guardian
 Posted: 12 Mar 2009 04:22 GMT Total Posts: 1111 | Both cases have to do with byte counts. Take this: Lbl A Goto A It has four bytes. The following functions identically but only uses three:While 1 End Similarly, DelVaring a variable only takes two bytes, whereas assigning any value to it requires at least three. In addition to consuming less memory, code that contains optimizations such as these generally execute faster.
--- -quoted directly from most movies that don't exist (and some that do). |
Vectris Ultralisk
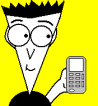 Posted: 12 Mar 2009 19:21 GMT Total Posts: 375 | Well While can't always do the same thing Gotos can, so I'd think they'd still be necessary.
As for the DelVar I tested it and got that DelVar X is the same number of bytes as 0->X. |
haveacalc Guardian
 Posted: 13 Mar 2009 10:55 GMT Total Posts: 1111 | Ah, I had forgotten that DelVar is a two-byte token. As far as the superiority of loops over labels, you'll find in object-oriented programming almost always calls for the former over the latter. Gotos are useful when the point in the program that they jump to is variable, which TI BASIC doesn't allow (unless you're on a 68k calc).
--- -quoted directly from most movies that don't exist (and some that do). |
Xphoenix Ultralisk
 Posted: 14 Mar 2009 11:27 GMT Total Posts: 210 | If the variable exists, 0→ is faster. If it doesn't, DelVar is faster.
DelVar does not update Ans, so you can use it instead of 0→[variable] if you want to keep the value of Ans.
DelVar actually deletes [variable] from memory, freeing up RAM, whereas 0→[variable] doesn't.
DelVar [variable] also let's you use commands without a colon:
:DelVar ADisp "HI is valid syntax.
:0→ADisp "HI is not.
--- ~Xphoenix |
me2 Goliath
 Posted: 16 Mar 2009 12:20 GMT Total Posts: 171 | You can decrease the size of your keypress routines by doing the following:
Using K to detect keypresses, x is horizontal coordinate, y is vertical coordinate: 3->X:3->Y Output(Y,X,* Lbl 1 0->K While K=0 getKey->K End Y-(K=25)+(K=34)->Y X-(K=24)+(K=26)->X ClrHome Output(Y,X,* Goto 1 End This will move a multiplication symbol around the screen. You must enter the two big store commands exactly as shown, you cannot change the signs unless you want to invert the controls. It is supposed to be -(K=25) and +(K=34). It is best to use a label for this routine, but only one is necessary. Note that for detection of any other keypress you can do a similar thing, but it is not the most efficient method. For anything other than the arrow keys, it is best to use an If statement.
--- <--- Going out with a bang. |
Vectris Ultralisk
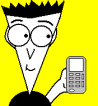 Posted: 16 Mar 2009 15:15 GMT Total Posts: 375 | To add onto the previous, you will almost always have to limit the movement, so you can place the restrictions right in with the key detection.
Y-(K=25 and Y<>0)+(K=34 and Y<>8)->Y
Where <> is not equal to, you can do the same for X. |
me2 Goliath
 Posted: 17 Mar 2009 07:21 GMT Total Posts: 171 | That's true, I didn't think of that but you should definitely include his idea.
--- <--- Going out with a bang. |
darksideprogramming Guardian
 Posted: 18 Mar 2009 23:37 GMT Total Posts: 1005 | I'm sure calcproductions meant:
Y-(K=25 and Y<>0)+(K=34 and Y<>8->Y
|
Vectris Ultralisk
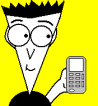 Posted: 19 Mar 2009 17:44 GMT Total Posts: 375 | *rolls eyes*
Ok ok, I forgot to take off the end parenthesis, now you can shoot me. |
haveacalc Guardian
 Posted: 19 Mar 2009 17:49 GMT Total Posts: 1111 | *optimizes bullet path*
--- -quoted directly from most movies that don't exist (and some that do). |